React
React projects created using Create React App or Vite should work out of the box with Preview.js, even if you use CSS-in-JS libraries such as Styled Components or Emotion.
You can confirm this by testing Preview.js with a simple <HelloWorld />
component:
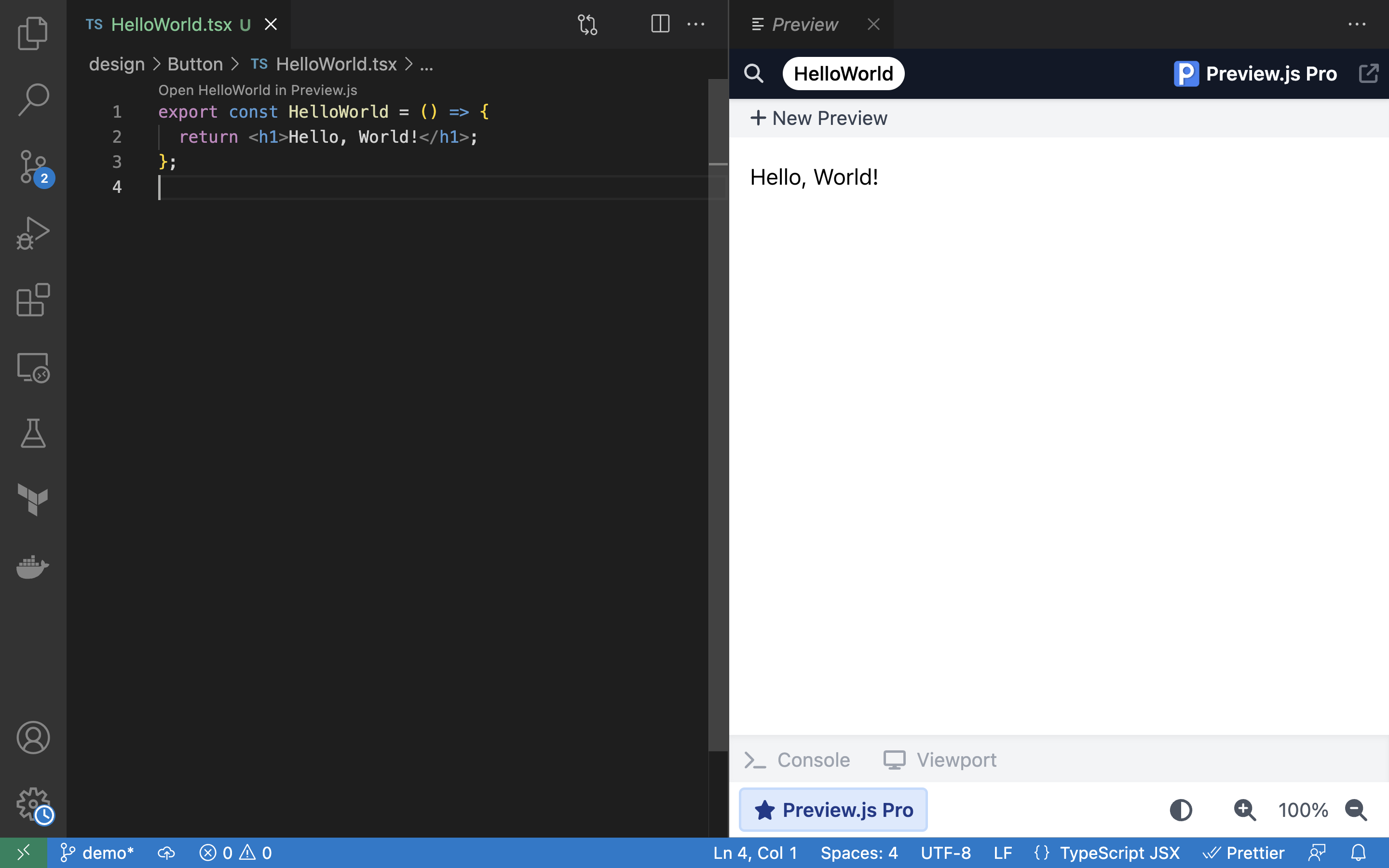
Assuming this works fine, it's time to try Preview.js with other components!
It's important to understand that not every component will preview successfully, because Preview.js renders them in isolation.
If you try to preview a component that depends on a particular React context or some other global state, it will
crash unless you set up a <Wrapper />
component to mock the required parts of your app and set up context providers.
Here are some common examples. You may need to combine them if you use several of these libraries (e.g. React Router + Redux).
Usage with React Router v5
// __previewjs__/Wrapper.tsx
import React from "react";
import { BrowserRouter } from "react-router-dom";
import "../src/index.css";
export const Wrapper = ({ children }) => (
<BrowserRouter>
{children}
</BrowserRouter>
);
Usage with React Router v6
// __previewjs__/Wrapper.tsx
import React from "react";
import { RouterProvider, createBrowserRouter } from "react-router-dom";
import "../src/index.css";
export const Wrapper = ({ children }) => (
<RouterProvider router={createBrowserRouter([{path: '*', element: children}])} />
);
Using Redux
// __previewjs__/Wrapper.tsx
import React from "react";
import { Provider } from "react-redux";
import store from "../src/redux";
import "../src/index.css";
export const Wrapper = ({ children }) => (
<Provider store={store}>
{children}
</Provider>
);